Sponsored Links
Ad by Google
We have already seen the implementation of one-to-one association in hibernate. In this post we are going to implement one-to-many association mapping example using hbm.xml file. One to many association means each row of a table can be related to many rows in the relating tables.
For example, we have an author and book table, we all know that a particular book is written by a particular author. An author of a book is always one and only one person, co-author of a book may be multiple but author always only one. So many books can be written by an author, here many books an author relationship is one-to-many association example.
author 1------------------------* books
Here is the example of author and book table of our one-to-many association example.
Author table:
Book table:
From the above two tables we have seen that author id 101 has written two books(1003,1004) and author 102 is also written two books(1001,1002) but the same book is not written by each other. So it is clear that an author has many books. Lets start implementation of these in hibernate practically.
Here is an One-To-Many association mapping of author and book table ER diagram:
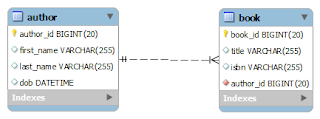
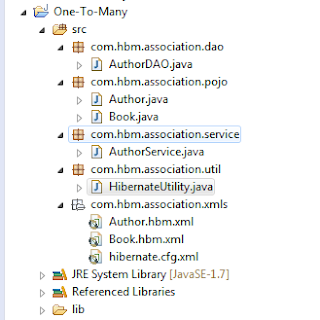
Main Objects of this project are:
Step 1. Database Script:
Step 2. Create a Java Project:
Go to File Menu then New->Java Project, and provide the name of the project.
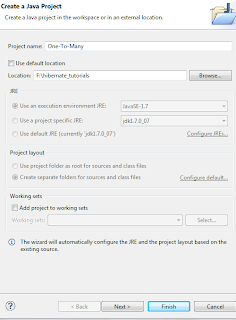
Create a lib folder(Optional):
Right click on your project and create a new folder named it as lib,this step is optional this is just to place all the required jar file. You may put all the jar files anywhere in your system's location, In this project we have placed all the required jar files in lib folder.
Step 3. Add libraries(jar files) into project's build path:
required jar files:
Step 4. Create a hibernate.cfg.xml file: Here we placed hibernate.cfg.xml file inside com.hbm.association.xmls folder.
hibernate.cfg.xml
Step 5. Create Author.hbm.xml file: Create a mapping file for author table.
Author.hbm.xml
Step 6. Create Book.hbm.xml file: Create a mapping file for book table.
Book.hbm.xml
Step 7. Create an Author Pojo class: Author class has all the properties mapped in Author.hbm.xml
Author.java
Step 8. Create a Book.java Pojo class: Book class has all the properties mapped in Book.hbm.xml
Book.java
Step 9. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration.
HibernateUtility.java
Step 10. Create an AuthorDAO class: AuthorDAO class to perform insert/update/get/delete records into database.
AuthorDAO.java
Step 11. Create an AuthorService class: AuthorService class to call the methods of AuthorDAO class.
AuthorService.java
That's it our one to many association example end here. Things to keep in mind while implementing one to many association is inverse attribute. We use inverse="true" in Author.hbm.xml file inverse attribute is always use in one to many or many to many association. inverse is use to assign the relationship owner, who will be the owner of relationship while implementing one to many or many to many association. Here inverse=true means Book is the relationship owner to maintain the relationship, so author is not going to call the update statements on book entity. Please see more on inverse example
Run the AuthorService class it will print 3 insert statement on your console, two for Book table and one for Author. Here we save author only but insert statements for book is also created because of cascade="all" in Author.hbm.xml for more on cascade example
OUT PUT:
Hibernate: insert into author (first_name, last_name, dob) values (?, ?, ?)
Hibernate: insert into book (title, isbn, author_id) values (?, ?, ?)
Hibernate: insert into book (title, isbn, author_id) values (?, ?, ?)
For required jar files you can download it from hibernate.org or from my previous post, here
For example, we have an author and book table, we all know that a particular book is written by a particular author. An author of a book is always one and only one person, co-author of a book may be multiple but author always only one. So many books can be written by an author, here many books an author relationship is one-to-many association example.
author 1------------------------* books
Here is the example of author and book table of our one-to-many association example.
Author table:
author_id | first_name | last_name | dob |
---|---|---|---|
101 | Joshua | Bloch | 1961-08-28 |
102 | Watts | S. Humphrey | 1927-07-04 |
Book table:
book_Id | title | isbn | author_id |
---|---|---|---|
1001 | A Discipline for Software Engineering | 0201546108 | 102 |
1002 | Managing the Software Process | 8177583301 | 102 |
1003 | Effective Java | 9780321356680 | 101 |
1004 | Java Puzzlers | 032133678X | 101 |
From the above two tables we have seen that author id 101 has written two books(1003,1004) and author 102 is also written two books(1001,1002) but the same book is not written by each other. So it is clear that an author has many books. Lets start implementation of these in hibernate practically.
Here is an One-To-Many association mapping of author and book table ER diagram:
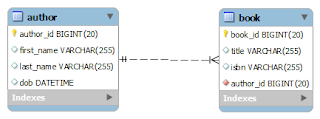
Tools and Technologies we are using here:
- JDK 7
- Hibernate 4.2.4
- MySql 5.5
- Eclipse Juno 4.2
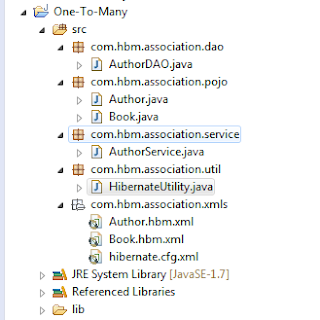
Main Objects of this project are:
- jar files
- hibernate.cfg.xml
- *hbm.xml (mapping files)
- database
Step 1. Database Script:
CREATE DATABASE /*!32312 IF NOT EXISTS*/`hibernate_tutorial`; USE `hibernate_tutorial`; /*Table structure for table `author` */ DROP TABLE IF EXISTS `author`; CREATE TABLE `author` ( `author_id` bigint(20) NOT NULL AUTO_INCREMENT, `first_name` varchar(255) DEFAULT NULL, `last_name` varchar(255) DEFAULT NULL, `dob` datetime DEFAULT NULL, PRIMARY KEY (`author_id`) ) ENGINE=InnoDB AUTO_INCREMENT=101 DEFAULT CHARSET=latin1; /*Table structure for table `book` */ DROP TABLE IF EXISTS `book`; CREATE TABLE `book` ( `book_id` bigint(20) NOT NULL AUTO_INCREMENT, `title` varchar(255) DEFAULT NULL, `isbn` varchar(255) DEFAULT NULL, `author_id` bigint(20) NOT NULL, PRIMARY KEY (`book_id`), KEY `FK_4sac2ubmnqva85r8bk8fxdvbf` (`author_id`), CONSTRAINT `FK_4sac2ubmnqva85r8bk8fxdvbf` FOREIGN KEY (`author_id`) REFERENCES `author` (`author_id`) ) ENGINE=InnoDB AUTO_INCREMENT=1001 DEFAULT CHARSET=latin1;
Step 2. Create a Java Project:
Go to File Menu then New->Java Project, and provide the name of the project.
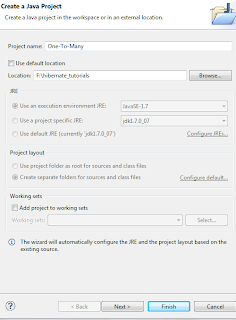
Create a lib folder(Optional):
Right click on your project and create a new folder named it as lib,this step is optional this is just to place all the required jar file. You may put all the jar files anywhere in your system's location, In this project we have placed all the required jar files in lib folder.
Step 3. Add libraries(jar files) into project's build path:
required jar files:
- antlr-2.7.7.jar
- commons-collections-3.2.1.jar
- dom4j-1.6.1.jar
- hibernate-commons-annotations-4.0.5.Final.jar
- hibernate-core-4.2.4.Final.jar
- hibernate-jpa-2.1-api-1.0.0.Final.jar
- jandex-1.1.0.Final.jar
- javassist-3.18.1-GA.jar
- jboss-logging-3.1.3.GA.jar
- jboss-transaction-api_1.1_spec-1.0.0.Final.jar
- mysql-connector-java-5.1.3-rc-bin.jar
Step 4. Create a hibernate.cfg.xml file: Here we placed hibernate.cfg.xml file inside com.hbm.association.xmls folder.
hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/hibernate_tutorial</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <!-- SQL dialect --> <property name="dialect">org.hibernate.dialect.MySQLDialect</property> <!-- Echo all executed SQL to stdout --> <property name="show_sql">true</property> <mapping resource="com/hbm/association/xmls/Author.hbm.xml"/> <mapping resource="com/hbm/association/xmls/Book.hbm.xml"/> </session-factory> </hibernate-configuration>
Step 5. Create Author.hbm.xml file: Create a mapping file for author table.
Author.hbm.xml
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <!-- Generated 29 Oct, 2014 8:47:37 PM by Hibernate Tools 3.4.0.CR1 --> <hibernate-mapping> <class name="com.hbm.association.pojo.Author" table="author"> <id name="authorId" column="author_id"> <generator class="identity" /> </id> <property name="firstName" column="first_name"/> <property name="lastName" column="last_name"/> <property name="dateOfBirth" column="dob" type="java.util.Date"/> <set name="books" table="book" inverse="true" cascade="all"> <key> <column name="author_id" not-null="true" /> </key> <one-to-many class="com.hbm.association.pojo.Book" /> </set> </class> </hibernate-mapping>
Step 6. Create Book.hbm.xml file: Create a mapping file for book table.
Book.hbm.xml
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <!-- Generated 29 Oct, 2014 8:47:37 PM by Hibernate Tools 3.4.0.CR1 --> <hibernate-mapping> <class name="com.hbm.association.pojo.Book" table="book"> <id name="bookId" column="book_id"> <generator class="identity" /> </id> <property name="title" column="title"/> <property name="isbn" column="isbn"/> <many-to-one name="author" class="com.hbm.association.pojo.Author"> <column name="author_id" not-null="true" /> </many-to-one> </class> </hibernate-mapping>
Step 7. Create an Author Pojo class: Author class has all the properties mapped in Author.hbm.xml
Author.java
package com.hbm.association.pojo; import java.util.Date; import java.util.HashSet; import java.util.Set; public class Author { private long authorId; private String firstName; private String lastName; private Date dateOfBirth; private Set<Book> books = new HashSet<>(); public long getAuthorId() { return authorId; } public void setAuthorId(long authorId) { this.authorId = authorId; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public Date getDateOfBirth() { return dateOfBirth; } public void setDateOfBirth(Date dateOfBirth) { this.dateOfBirth = dateOfBirth; } public Set<Book> getBooks() { return books; } public void setBooks(Set<Book> books) { this.books = books; } }
Step 8. Create a Book.java Pojo class: Book class has all the properties mapped in Book.hbm.xml
Book.java
package com.hbm.association.pojo; public class Book { private long bookId; private String title; private String isbn; private Author author; public long getBookId() { return bookId; } public void setBookId(long bookId) { this.bookId = bookId; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getIsbn() { return isbn; } public void setIsbn(String isbn) { this.isbn = isbn; } public Author getAuthor() { return author; } public void setAuthor(Author author) { this.author = author; } }
Step 9. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration.
HibernateUtility.java
package com.hbm.association.util; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; import org.hibernate.service.ServiceRegistryBuilder; public class HibernateUtility { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure("/com/hbm/association/xmls/hibernate.cfg.xml"); ServiceRegistry serviceRegistry = new ServiceRegistryBuilder(). applySettings(configuration.getProperties()).buildServiceRegistry(); SessionFactory sessionFactory = configuration.buildSessionFactory(serviceRegistry); return sessionFactory; } public static SessionFactory getSessionFactory() { return sessionFactory; } }
Step 10. Create an AuthorDAO class: AuthorDAO class to perform insert/update/get/delete records into database.
AuthorDAO.java
package com.hbm.association.dao; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.hbm.association.pojo.Author; import com.hbm.association.util.HibernateUtility; public class AuthorDAO { private static SessionFactory sessionFactory; static { sessionFactory = HibernateUtility.getSessionFactory(); } public static Author findById(long id) { Session session = sessionFactory.openSession(); Author author = (Author) session.get(Author.class, id); return author; } public static Author save(Author author) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.save(author); session.getTransaction().commit(); return author; } public static Author update(Author author) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.merge(author); session.getTransaction().commit(); return author; } public static void delete(Author author) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.delete(author); session.getTransaction().commit(); } }
Step 11. Create an AuthorService class: AuthorService class to call the methods of AuthorDAO class.
AuthorService.java
package com.hbm.association.service; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.HashSet; import java.util.Set; import com.hbm.association.dao.AuthorDAO; import com.hbm.association.pojo.Author; import com.hbm.association.pojo.Book; public class AuthorService { public static void main(String[] args) throws ParseException { Author author = new Author(); author.setFirstName("Joshua"); author.setLastName("Bloch"); SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); author.setDateOfBirth(sdf.parse("1961-08-28")); Book effectiveJava = new Book(); effectiveJava.setTitle("Effective Java"); effectiveJava.setIsbn("9780321356680"); effectiveJava.setAuthor(author); Book javaPuzzlers = new Book(); javaPuzzlers.setTitle("Java Puzzlers"); javaPuzzlers.setIsbn("032133678X"); javaPuzzlers.setAuthor(author); Set<Book> books = new HashSet<>(); books.add(javaPuzzlers); books.add(effectiveJava); author.setBooks(books); AuthorDAO.save(author); } }
That's it our one to many association example end here. Things to keep in mind while implementing one to many association is inverse attribute. We use inverse="true" in Author.hbm.xml file inverse attribute is always use in one to many or many to many association. inverse is use to assign the relationship owner, who will be the owner of relationship while implementing one to many or many to many association. Here inverse=true means Book is the relationship owner to maintain the relationship, so author is not going to call the update statements on book entity. Please see more on inverse example
Run the AuthorService class it will print 3 insert statement on your console, two for Book table and one for Author. Here we save author only but insert statements for book is also created because of cascade="all" in Author.hbm.xml for more on cascade example
OUT PUT:
Hibernate: insert into author (first_name, last_name, dob) values (?, ?, ?)
Hibernate: insert into book (title, isbn, author_id) values (?, ?, ?)
Hibernate: insert into book (title, isbn, author_id) values (?, ?, ?)
Download the Complete example from here Source Code
For required jar files you can download it from hibernate.org or from my previous post, here
Sponsored Links
0 comments:
Post a Comment