Sponsored Links
Ad by Google
We have already seen the implementation of one-to-one association in hibernate. In this post we are going to implement One-To-Many association mapping example using annotation. For One-To-Many association example using hbm.xml file see our previous article One-To-Many example using hbm.xml
One to many association means each row of a table can be related to many rows in the relating tables.
For example, we have an author and book table, we all know that a particular book is written by a particular author. An author of a book is always one and only one person, co-author of a book may be multiple but author always only one. So many books can be written by an author, here many books an author relationship is one-to-many association example.
an author has many books that is. 1------------------------* books( one-to-may association)
Here is the example of author and book table for our One-To-Many association example.
Author table:
Book table:
From the above two tables we have seen that author id 101 has written two books(1003,1004) and author 102 is also written two books(1001,1002) but the same book is not written by each other. So it is clear that an author has many books. Lets start implementation of these in hibernate practically.
Here is an One-To-Many association mapping of an author and book table ER diagram:
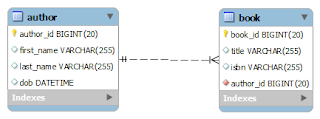

Main Objects of this project are:
Step 1. Database Script:
Step 2. Create a Maven Project:
Step A: Go to File->New->Other..
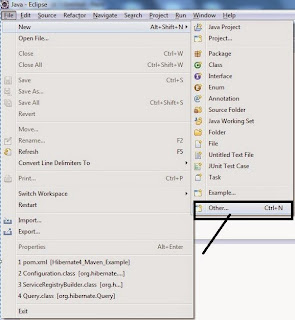
Step B: Select Maven Project from the select wizard.
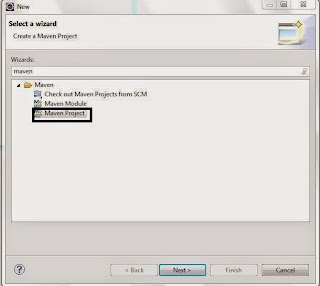
Step C: Select project name and location from New Maven Project wizard.
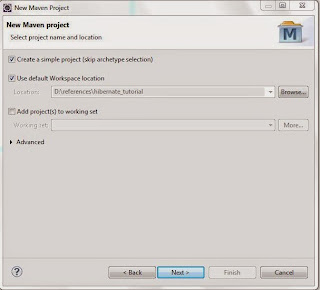
Step D: Configure project, provide GroupId, artifactId etc. See the details from the screenshot. This screen shot is from our previous post so here you need to change the artifactId as One-To-Many-Association.
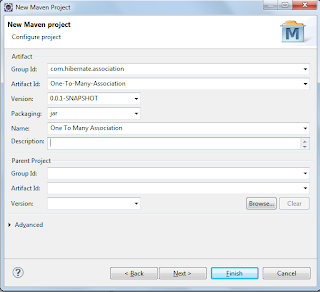
Step E: After completion of all the above steps, now your project will looks like this screenshot.

3. Add project dependencies into pom.xml file:
Double click on your project's pom.xml file it will looks like this with very limited information.
Now add hibernate and mysql dependencies entry inside pom.xml file. Paste the below code inside the project tag of pom.xml file.
Here is a complete pom.xml file
4. Create a hibernate.cfg.xml file:
Create hibernate.cfg.xml file inside src/main/resources folder.
hibernate.cfg.xml
Step 5. Create an Author annotated class:
Step 6. Create a Book annotated class
Step 7. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration file.
HibernateUtility .java
Step 8. Create an AuthorDAO class: to perform insert/update/get/delete records into the database.
Step 9. Create an AuthorService class: to call the methods of AuthorDAO class to perform CRUD.
That's it our one to many association example end here. Run the AuthorService class it will print 3 insert statement on your console, two for Book table and one for Author. Here we save author only but insert statements for book is also created because of cascade=CascadeType.ALL in Author.java class.
OUT PUT:
Hibernate: insert into author (first_name, last_name, dob) values (?, ?, ?)
Hibernate: insert into book (title, isbn, author_id) values (?, ?, ?)
Hibernate: insert into book (title, isbn, author_id) values (?, ?, ?)
You can download the same application using hbm.xml without using maven from here One-To-Many association using hbm.xml
One to many association means each row of a table can be related to many rows in the relating tables.
For example, we have an author and book table, we all know that a particular book is written by a particular author. An author of a book is always one and only one person, co-author of a book may be multiple but author always only one. So many books can be written by an author, here many books an author relationship is one-to-many association example.
an author has many books that is. 1------------------------* books( one-to-may association)
Here is the example of author and book table for our One-To-Many association example.
Author table:
author_id | first_name | last_name | dob |
---|---|---|---|
101 | Joshua | Bloch | 1961-08-28 |
102 | Watts | S. Humphrey | 1927-07-04 |
Book table:
book_Id | title | isbn | author_id |
---|---|---|---|
1001 | A Discipline for Software Engineering | 0201546108 | 102 |
1002 | Managing the Software Process | 8177583301 | 102 |
1003 | Effective Java | 9780321356680 | 101 |
1004 | Java Puzzlers | 032133678X | 101 |
From the above two tables we have seen that author id 101 has written two books(1003,1004) and author 102 is also written two books(1001,1002) but the same book is not written by each other. So it is clear that an author has many books. Lets start implementation of these in hibernate practically.
Here is an One-To-Many association mapping of an author and book table ER diagram:
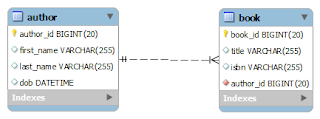
Tools and Technologies we are using here:
- JDK 7
- Hibernate 4.2.4
- MySql 5.1.10
- Eclipse Juno 4.2

Main Objects of this project are:
- pom.xml
- hibernate.cfg.xml
- annotated pojo
- database
Step 1. Database Script:
CREATE DATABASE /*!32312 IF NOT EXISTS*/`hibernate_tutorial`; USE `hibernate_tutorial`; /*Table structure for table `author` */ DROP TABLE IF EXISTS `author`; CREATE TABLE `author` ( `author_id` bigint(20) NOT NULL AUTO_INCREMENT, `first_name` varchar(255) DEFAULT NULL, `last_name` varchar(255) DEFAULT NULL, `dob` datetime DEFAULT NULL, PRIMARY KEY (`author_id`) ) ENGINE=InnoDB AUTO_INCREMENT=101 DEFAULT CHARSET=latin1; /*Table structure for table `book` */ DROP TABLE IF EXISTS `book`; CREATE TABLE `book` ( `book_id` bigint(20) NOT NULL AUTO_INCREMENT, `title` varchar(255) DEFAULT NULL, `isbn` varchar(255) DEFAULT NULL, `author_id` bigint(20) NOT NULL, PRIMARY KEY (`book_id`), KEY `FK_4sac2ubmnqva85r8bk8fxdvbf` (`author_id`), CONSTRAINT `FK_4sac2ubmnqva85r8bk8fxdvbf` FOREIGN KEY (`author_id`) REFERENCES `author` (`author_id`) ) ENGINE=InnoDB AUTO_INCREMENT=1001 DEFAULT CHARSET=latin1;
Step 2. Create a Maven Project:
Step A: Go to File->New->Other..
Step B: Select Maven Project from the select wizard.
Step C: Select project name and location from New Maven Project wizard.
Step D: Configure project, provide GroupId, artifactId etc. See the details from the screenshot. This screen shot is from our previous post so here you need to change the artifactId as One-To-Many-Association.
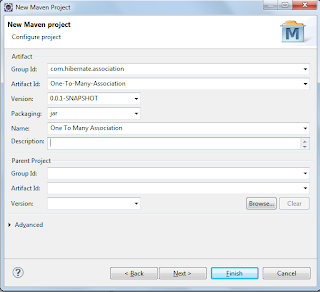
Step E: After completion of all the above steps, now your project will looks like this screenshot.

3. Add project dependencies into pom.xml file:
Double click on your project's pom.xml file it will looks like this with very limited information.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.hibernate.association</groupId> <artifactId>One-To-Many-Association</artifactId> <version>0.0.1-SNAPSHOT</version> <name>One-To-Many-Association</name> </project>
Now add hibernate and mysql dependencies entry inside pom.xml file. Paste the below code inside the project tag of pom.xml file.
<dependencies> <!-- Hibernate Dependency --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>4.2.4.Final</version> </dependency> <!-- MySql Connector dependency --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.10</version> </dependency> </dependencies>
Here is a complete pom.xml file
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.hibernate.association</groupId> <artifactId>One-To-Many-Association</artifactId> <version>0.0.1-SNAPSHOT</version> <name>One-To-Many-Association</name> <dependencies> <!-- Hibernate Dependency --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>4.2.4.Final</version> </dependency> <!-- MySql Connector dependency --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.10</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.7</source> <target>1.7</target> </configuration> </plugin> </plugins> </build> </project>
4. Create a hibernate.cfg.xml file:
Create hibernate.cfg.xml file inside src/main/resources folder.
hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/hibernate_tutorial</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <!-- SQL dialect --> <property name="dialect">org.hibernate.dialect.MySQLDialect</property> <!-- Echo all executed SQL to stdout --> <property name="show_sql">true</property> <property name="hbm2ddl.auto">update</property> <mapping class="com.hibernate.association.pojo.Author"/> <mapping class="com.hibernate.association.pojo.Book"/> </session-factory> </hibernate-configuration>
Step 5. Create an Author annotated class:
package com.hibernate.association.pojo; import java.util.Date; import java.util.HashSet; import java.util.Set; import javax.persistence.CascadeType; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.OneToMany; import javax.persistence.Table; import javax.persistence.Temporal; import javax.persistence.TemporalType; @Entity @Table(name="author") public class Author { @Id @GeneratedValue(strategy=GenerationType.IDENTITY) @Column(name="author_id", unique=true, nullable=false) private long authorId; @Column(name="first_name") private String firstName; @Column(name="last_name") private String lastName; @Temporal(TemporalType.DATE) @Column(name="dob") private Date dateOfBirth; @OneToMany(mappedBy="author",cascade=CascadeType.ALL) private Set<Book> books = new HashSet<>(); public long getAuthorId() { return authorId; } public void setAuthorId(long authorId) { this.authorId = authorId; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public Date getDateOfBirth() { return dateOfBirth; } public void setDateOfBirth(Date dateOfBirth) { this.dateOfBirth = dateOfBirth; } public Set<Book> getBooks() { return books; } public void setBooks(Set<Book> books) { this.books = books; } }
Step 6. Create a Book annotated class
package com.hibernate.association.pojo; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.JoinColumn; import javax.persistence.ManyToOne; import javax.persistence.Table; @Entity @Table(name="book") public class Book { @Id @GeneratedValue(strategy=GenerationType.IDENTITY) @Column(name="book_id", unique=true, nullable=false) private long bookId; @Column(name="title") private String title; @Column(name = "isbn") private String isbn; @ManyToOne @JoinColumn(name = "author_id", nullable=false) private Author author; public long getBookId() { return bookId; } public void setBookId(long bookId) { this.bookId = bookId; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getIsbn() { return isbn; } public void setIsbn(String isbn) { this.isbn = isbn; } public Author getAuthor() { return author; } public void setAuthor(Author author) { this.author = author; } }
Step 7. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration file.
HibernateUtility .java
package com.hibernate.association.util; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; import org.hibernate.service.ServiceRegistryBuilder; public class HibernateUtility { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure(); ServiceRegistry serviceRegistry = new ServiceRegistryBuilder(). applySettings(configuration.getProperties()).buildServiceRegistry(); SessionFactory sessionFactory = configuration.buildSessionFactory(serviceRegistry); return sessionFactory; } public static SessionFactory getSessionFactory() { return sessionFactory; } }
Step 8. Create an AuthorDAO class: to perform insert/update/get/delete records into the database.
package com.hibernate.association.dao; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.hibernate.association.pojo.Author; import com.hibernate.association.util.HibernateUtility; public class AuthorDAO { private static SessionFactory sessionFactory; static { sessionFactory = HibernateUtility.getSessionFactory(); } public static Author findById(long id) { Session session = sessionFactory.openSession(); Author author = (Author) session.load(Author.class, id); return author; } public static Author save(Author author) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.save(author); session.getTransaction().commit(); return author; } public static Author update(Author author) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.merge(author); session.getTransaction().commit(); return author; } public static void delete(Author author) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.delete(author); session.getTransaction().commit(); } }
Step 9. Create an AuthorService class: to call the methods of AuthorDAO class to perform CRUD.
package com.hibernate.association.service; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.HashSet; import java.util.Set; import com.hibernate.association.dao.AuthorDAO; import com.hibernate.association.pojo.Author; import com.hibernate.association.pojo.Book; public class AuthorService { public static void main(String[] args) throws ParseException { Author author = new Author(); author.setFirstName("Joshua"); author.setLastName("Bloch"); SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); author.setDateOfBirth(sdf.parse("1961-08-28")); // book 1 Book effectiveJava = new Book(); effectiveJava.setTitle("Effective Java"); effectiveJava.setIsbn("9780321356680"); effectiveJava.setAuthor(author); // book 2 Book javaPuzzlers = new Book(); javaPuzzlers.setTitle("Java Puzzlers"); javaPuzzlers.setIsbn("032133678X"); javaPuzzlers.setAuthor(author); Set<Book> books = new HashSet<>(); books.add(javaPuzzlers); books.add(effectiveJava); author.setBooks(books); AuthorDAO.save(author); } }
That's it our one to many association example end here. Run the AuthorService class it will print 3 insert statement on your console, two for Book table and one for Author. Here we save author only but insert statements for book is also created because of cascade=CascadeType.ALL in Author.java class.
OUT PUT:
Hibernate: insert into author (first_name, last_name, dob) values (?, ?, ?)
Hibernate: insert into book (title, isbn, author_id) values (?, ?, ?)
Hibernate: insert into book (title, isbn, author_id) values (?, ?, ?)
Download the complete example from here Source Code
You can download the same application using hbm.xml without using maven from here One-To-Many association using hbm.xml
Sponsored Links
thanks
ReplyDelete