Sponsored Links
Ad by Google
In this post we are going to create a very basic java CRUD application using hibernate to insert/update/select/delete records into MySql database. In this post we are using hibernate vi .hbm.xml file based not annotation based. In this project we have a single table called user and we are going to play with user table only.
Technologies/Tools we are used here:
Our project will looks like this:
To create a very basic hibernate crud application using hbm.xml file we need
Here is a user table with very few columns:
1. Create table query:
Technologies/Tools we are used here:
- JDK 7
- Hibernate 4.0
- MySql 5.0
- Eclipse Juno
project structure |
To create a very basic hibernate crud application using hbm.xml file we need
- jar files
- hibernate.cfg.xml
- *.hbm.xml
- database
Here is a user table with very few columns:
1. Create table query:
/*Table structure for table `user` */ DROP TABLE IF EXISTS `user`; CREATE TABLE `user` ( `id` int(11) NOT NULL AUTO_INCREMENT, `user_name` varchar(50) DEFAULT NULL, `password` varchar(20) DEFAULT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB AUTO_INCREMENT=9 DEFAULT CHARSET=latin1;
2. Create a Java project
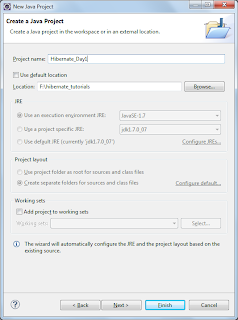
Create lib folder (Optional):
Right click on your project and create a new folder named it as lib,this step is optional this is just to place all the jars here. You may put all the jars anywhere in your system's location, In this project we are putting all the jar files in lib folder.
3. Add libraries(jars) into project's build path.
required jar files:
- antlr-2.7.7.jar
- commons-collections-3.2.1.jar
- dom4j-1.6.1.jar
- hibernate-commons-annotations-4.0.1.Final.jar
- hibernate-core-4.0.0.Final.jar
- hibernate-jpa-2.0-api-1.0.1.Final.jar
- javassist-3.12.1.GA.jar
- jboss-logging-3.1.0.CR2.jar
- jboss-transaction-api_1.1_spec-1.0.0.Final.jar
- mysql-connector-java-5.1.3-rc-bin.jar
Right click on your project go to Build Path > Configure Build Path
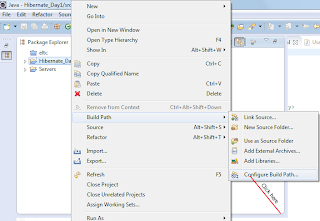
Now, Click on libraries, If you placed all the jars in lib folder of the project then click on Add Jars otherwise click on Add External JARs. and select the jar files.
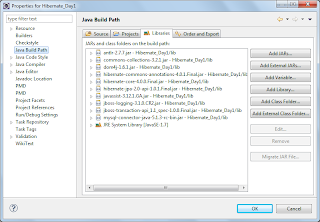
4. create a hibernate.cfg.xml file
Create a hibernate.cfg.xml configuration file inside your src folder. Minimum properties required inside hibernate.cfg.xml file are
- connection.driver_class
- connection.url
- connection.username
- connection.password
- dialect
- mapping resource
Here is a complete hibernate.cfg.xml file:
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/test</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <!-- SQL dialect --> <property name="dialect">org.hibernate.dialect.MySQLDialect</property> <!-- Echo all executed SQL to stdout --> <property name="show_sql">true</property> <mapping resource="com/hbm/xmls/User.hbm.xml"/> </session-factory> </hibernate-configuration>
5. Create User.hbm.xml
Now create User.hbm.xml file, we have a user table so we created User.hbm.xml file (not mandatory to create tablename.hbm.xml you may create xyz.hbm.xml also).
Here is a complete User.hbm.xml file:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping package="com.hbm.day1.pojo"> <class name="User" table="user"> <id name="userId" column="id"> <generator class="native"/> </id> <property name="userName" column="user_name" /> <property name="password" /> </class> </hibernate-mapping>
6. User class, which is a pojo class
User class is pojo class which has all the properties of user table we mapped in user.hbm.xml file.
User.java
package com.hbm.day1.pojo; public class User { private int userId; private String userName; private String password; public int getUserId() { return userId; } public void setUserId(int userId) { this.userId = userId; } public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } @Override public String toString() { return "User [userId=" + userId + ", userName=" + userName + ", password=" + password + "]"; } }
7. Create a Utility class to Build SessionFactory vi Loading Configuration
Here we are creating HibernateUtility class to build SessionFactory from the Configuration.
HibernateUtility .java
package com.hbm.util; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; import org.hibernate.service.ServiceRegistryBuilder; public class HibernateUtility { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure(); ServiceRegistry serviceRegistry = new ServiceRegistryBuilder(). applySettings(configuration.getProperties()).buildServiceRegistry(); SessionFactory sessionFactory = configuration.buildSessionFactory(serviceRegistry); return sessionFactory; } public static SessionFactory getSessionFactory() { return sessionFactory; } }
8. Create UserDAO class
Creating a UserDAO class to perform all the DAO related methods such as insert/update/select/delete records into MySql database.
UserDAO.java
package com.hbm.day1.dao; import java.util.List; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.hbm.day1.pojo.User; import com.hbm.util.HibernateUtility; public class UserDAO { private static SessionFactory sessionFactory; static { sessionFactory = HibernateUtility.getSessionFactory(); } public static User findById(int id) { Session session = sessionFactory.openSession(); User user = (User) session.get(User.class, id); return user; } public static User save(User user) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.save(user); session.getTransaction().commit(); return user; } public static User update(User user) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.merge(user); session.getTransaction().commit(); return user; } public static void delete(User user) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.delete(user); session.getTransaction().commit(); } @SuppressWarnings("unchecked") public static List<User> findAll(){ Session session = sessionFactory.openSession(); return session.createQuery("from User").list(); } }
9. Create UserService class to call these methods
Creating UserService class to call these methods UserService class has a main method.
UserService.java
package com.hbm.day1.service; import com.hbm.day1.dao.UserDAO; import com.hbm.day1.pojo.User; public class UserService { public static void main(String[] args) { User user =new User(); user.setPassword("xxxxxxxx"); user.setUserName("javamakeuse"); //calling save method. UserDAO.save(user); // calling findById. user = UserDAO.findById(1); System.out.println(user); user.setUserName("info@javamakeuse.com"); //calling update method. UserDAO.update(user); System.out.println(UserDAO.findById(1).getUserName()); // calling findAll method. System.out.println(UserDAO.findAll()); } }
When you run UserService, you will get this out put at your console.

Output:
Hibernate: insert into user (user_name, password) values (?, ?)
Hibernate: select user0_.id as id0_0_, user0_.user_name as user2_0_0_, user0_.password as password0_0_ from user user0_ where user0_.id=?
User [userId=1, userName=javamakeuse, password=xxxxxxxx]
Hibernate: select user0_.id as id0_0_, user0_.user_name as user2_0_0_, user0_.password as password0_0_ from user user0_ where user0_.id=?
Hibernate: update user set user_name=?, password=? where id=?
Hibernate: select user0_.id as id0_0_, user0_.user_name as user2_0_0_, user0_.password as password0_0_ from user user0_ where user0_.id=?
info@javamakeuse.com
Hibernate: select user0_.id as id0_, user0_.user_name as user2_0_, user0_.password as password0_ from user user0_
[User [userId=1, userName=info@javamakeuse.com, password=xxxxxxxx]]
Note: If you notice your console output, here it's printing sql statements also, because we are printing this using <property name="show_sql">true</property> in hibernate.cfg.xml file to hide this statements make it false from true or remove the complete line.
Download the Complete example from here Source Code + lib
Sponsored Links
0 comments:
Post a Comment