Sponsored Links
Ad by Google
In this post we are going to create a project to implement the step by step one-to-one association example using annotation with maven project. Before that lets have a look what exactly one-to-one association in RDBMS ? One-to-One association means, Each row of table is mapped with exactly one and only one row with another table.
For example, we have a passport_detail and person table each row of person table is mapped with exactly one and only one row of passport_detail table. One person has only one passport.
For one-to-one association example with hbm.xml file see this one-to-one association using hbm.xml post.
Here is an one-to-one association mapping of person and passport_detail table ER diagram:
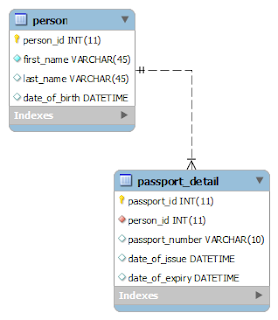
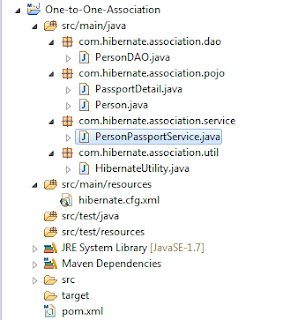
Main Objects of this project are:
Step 1. Database Script:
Step 2. Create a Maven Project:
Step A: Go to File->New->Other..
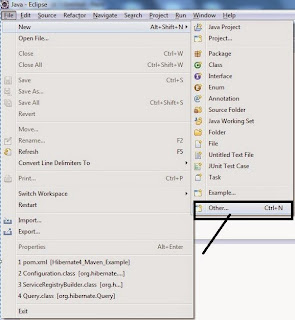
Step B: Select Maven Project from the select wizard.
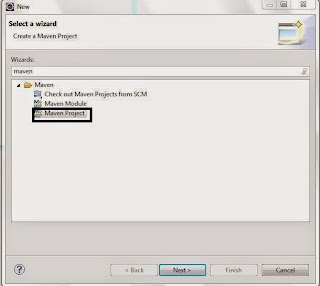
Step C: Select project name and location from New Maven Project wizard.
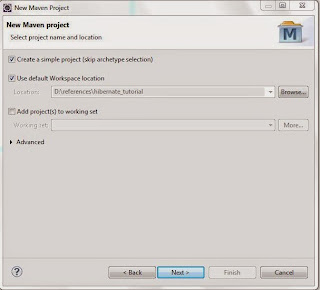
Step D: Configure project, provide GroupId, artifactId etc. See the details from the screenshot. This screen shot is from my previous post so here you need to change the artifactId as One-to-One-Association.
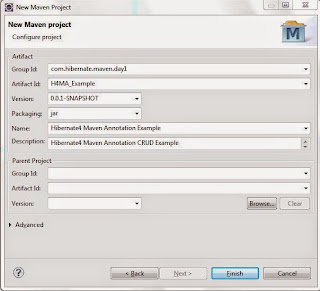
Step E: After completion of all the above steps, now your project will looks like this screenshot.
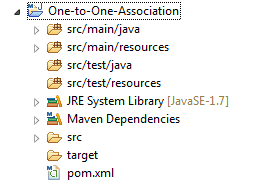
3. Add project dependencies into pom.xml file:
Double click on your project's pom.xml file it will looks like this with very limited information.
Now add hibernate and mysql dependencies entry inside pom.xml file. Paste the below code inside the project tag of pom.xml file.
Here is a complete pom.xml file
4. Create a hibernate.cfg.xml file:
Create hibernate.cfg.xml file inside src/main/resources folder.
hibernate.cfg.xml
Step 5. Create Person annotated class:
Step 6. Create PassportDetail annotated class:
Step 7. Create a HibernateUtility class: HibernateUtility class to build SessionFactory vi loading Configuration.
HibernateUtility .java
Step 8. Create PersonDAO class: PersonDAO class to perform insert/update/get/delete records into database.
PersonDAO.java
Step 9. Create PersonPassportService class: Service class to call the methods of PersonDAO class.
PersonPassportService.java
That's it, Now run the PersonPassportService class you will get output at your console where two insert query will executed one for Person and one for PasspportDetail table, along with passport details because we are overriding the toString() method inside Person.java class.
OUT PUT:
Hibernate: insert into person (date_of_birth, first_name, last_name) values (?, ?, ?)
Hibernate: insert into passport_detail (date_of_expiry, date_of_issue, passport_number, person_id) values (?, ?, ?, ?)
Person [personId=1, firstName=Tony, lastName=Leo, dateOfBirth=1990-10-10]
For example, we have a passport_detail and person table each row of person table is mapped with exactly one and only one row of passport_detail table. One person has only one passport.
For one-to-one association example with hbm.xml file see this one-to-one association using hbm.xml post.
Here is an one-to-one association mapping of person and passport_detail table ER diagram:
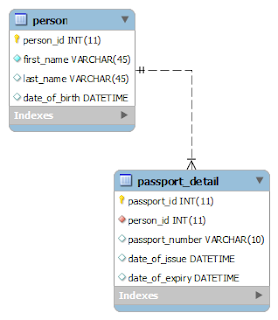
Tools and Technologies we are using here:
- JDK 7
- Maven 3.2.2
- Hibernate 4.2.4
- MySql 5.5
- Eclipse Juno 4.2
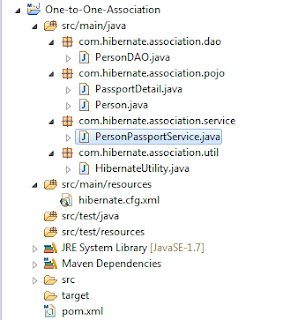
Main Objects of this project are:
- pom.xml
- hibernate.cfg.xml
- annotated pojo
- database
Step 1. Database Script:
CREATE DATABASE `hibernate_tutorial` ; USE `hibernate_tutorial`; /*Table structure for table `passport_detail` */ DROP TABLE IF EXISTS `passport_detail`; CREATE TABLE `passport_detail` ( `passport_id` int(11) NOT NULL AUTO_INCREMENT, `date_of_expiry` date DEFAULT NULL, `date_of_issue` date DEFAULT NULL, `passport_number` varchar(255) DEFAULT NULL, `person_id` int(11) DEFAULT NULL, PRIMARY KEY (`passport_id`), KEY `FK_5rxo4attorxuavdigd8k9ysf8` (`person_id`), CONSTRAINT `FK_5rxo4attorxuavdigd8k9ysf8` FOREIGN KEY (`person_id`) REFERENCES `person` (`person_id`) ) ENGINE=InnoDB AUTO_INCREMENT=4 DEFAULT CHARSET=latin1; /*Table structure for table `person` */ DROP TABLE IF EXISTS `person`; CREATE TABLE `person` ( `person_id` int(11) NOT NULL AUTO_INCREMENT, `date_of_birth` date DEFAULT NULL, `first_name` varchar(255) DEFAULT NULL, `last_name` varchar(255) DEFAULT NULL, PRIMARY KEY (`person_id`) ) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=latin1;
Step 2. Create a Maven Project:
Step A: Go to File->New->Other..
Step B: Select Maven Project from the select wizard.
Step C: Select project name and location from New Maven Project wizard.
Step D: Configure project, provide GroupId, artifactId etc. See the details from the screenshot. This screen shot is from my previous post so here you need to change the artifactId as One-to-One-Association.
Step E: After completion of all the above steps, now your project will looks like this screenshot.
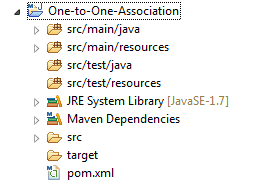
3. Add project dependencies into pom.xml file:
Double click on your project's pom.xml file it will looks like this with very limited information.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.hibernate.association</groupId> <artifactId>One-to-One-Association</artifactId> <version>0.0.1-SNAPSHOT</version> <name>One-to-One-Association</name> <description>One-to-One-Association example</description> </project>
Now add hibernate and mysql dependencies entry inside pom.xml file. Paste the below code inside the project tag of pom.xml file.
<dependencies> <!-- Hibernate Dependency --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>4.2.4.Final</version> </dependency> <!-- MySql Connector dependency --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.10</version> </dependency> </dependencies>
Here is a complete pom.xml file
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.hibernate.association</groupId> <artifactId>One-to-One-Association</artifactId> <version>0.0.1-SNAPSHOT</version> <name>One-to-One-Association</name> <description>One-to-One-Association example</description> <dependencies> <!-- Hibernate Dependency --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>4.2.4.Final</version> </dependency> <!-- MySql Connector dependency --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.10</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.7</source> <target>1.7</target> </configuration> </plugin> </plugins> </build> </project>
4. Create a hibernate.cfg.xml file:
Create hibernate.cfg.xml file inside src/main/resources folder.
hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/hibernate_tutorial</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <!-- SQL dialect --> <property name="dialect">org.hibernate.dialect.MySQLDialect</property> <!-- Echo all executed SQL to stdout --> <property name="show_sql">true</property> <property name="hbm2ddl.auto">update</property> <mapping class="com.hibernate.association.pojo.Person"/> <mapping class="com.hibernate.association.pojo.PassportDetail"/> </session-factory> </hibernate-configuration>
Step 5. Create Person annotated class:
package com.hibernate.association.pojo; import java.text.SimpleDateFormat; import java.util.Date; import javax.persistence.CascadeType; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.OneToOne; import javax.persistence.Table; import javax.persistence.Temporal; import javax.persistence.TemporalType; @Entity @Table(name="person") public class Person { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @Column(name = "person_id", unique = true, nullable = false) private Integer personId; @Column(name="first_name") private String firstName; @Column(name="last_name") private String lastName; @Temporal(TemporalType.DATE) @Column(name="date_of_birth") private Date dateOfBirth; @OneToOne(mappedBy = "person",cascade=CascadeType.ALL) private PassportDetail passportDetail; public Integer getPersonId() { return personId; } public void setPersonId(Integer personId) { this.personId = personId; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public Date getDateOfBirth() { return dateOfBirth; } public void setDateOfBirth(Date dateOfBirth) { this.dateOfBirth = dateOfBirth; } public PassportDetail getPassportDetail() { return passportDetail; } public void setPassportDetail(PassportDetail passportDetail) { this.passportDetail = passportDetail; } @Override public String toString() { return "Person [personId=" + personId + ", firstName=" + firstName + ", lastName=" + lastName + ", dateOfBirth=" + new SimpleDateFormat("yyyy-MM-dd").format(dateOfBirth) + "]"; } }
Step 6. Create PassportDetail annotated class:
package com.hibernate.association.pojo; import java.util.Date; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.JoinColumn; import javax.persistence.OneToOne; import javax.persistence.Table; import javax.persistence.Temporal; import javax.persistence.TemporalType; @Entity @Table(name = "passport_detail") public class PassportDetail { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @Column(name = "passport_id", unique = true, nullable = false) private Integer passportId; @Column(name = "passport_number") private String passportNo; @Temporal(TemporalType.DATE) @Column(name = "date_of_issue") private Date dateOfIssue; @Temporal(TemporalType.DATE) @Column(name = "date_of_expiry") private Date dateOfExpiry; @OneToOne @JoinColumn(name = "person_id") private Person person; public Integer getPassportId() { return passportId; } public void setPassportId(Integer passportId) { this.passportId = passportId; } public String getPassportNo() { return passportNo; } public void setPassportNo(String passportNo) { this.passportNo = passportNo; } public Date getDateOfIssue() { return dateOfIssue; } public void setDateOfIssue(Date dateOfIssue) { this.dateOfIssue = dateOfIssue; } public Date getDateOfExpiry() { return dateOfExpiry; } public void setDateOfExpiry(Date dateOfExpiry) { this.dateOfExpiry = dateOfExpiry; } public Person getPerson() { return person; } public void setPerson(Person person) { this.person = person; } @Override public String toString() { return "PassportDetail [passportId=" + passportId + ", passportNo=" + passportNo + ", dateOfIssue=" + dateOfIssue + ", dateOfExpiry=" + dateOfExpiry + ", person=" + person + "]"; } }
Step 7. Create a HibernateUtility class: HibernateUtility class to build SessionFactory vi loading Configuration.
HibernateUtility .java
package com.hibernate.association.util; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; import org.hibernate.service.ServiceRegistryBuilder; public class HibernateUtility { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure(); ServiceRegistry serviceRegistry = new ServiceRegistryBuilder(). applySettings(configuration.getProperties()).buildServiceRegistry(); SessionFactory sessionFactory = configuration.buildSessionFactory(serviceRegistry); return sessionFactory; } public static SessionFactory getSessionFactory() { return sessionFactory; } }
Step 8. Create PersonDAO class: PersonDAO class to perform insert/update/get/delete records into database.
PersonDAO.java
package com.hibernate.association.dao; import java.util.List; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.hibernate.association.pojo.Person; import com.hibernate.association.util.HibernateUtility; public class PersonDAO { private static SessionFactory sessionFactory; static { sessionFactory = HibernateUtility.getSessionFactory(); } public static Person findById(int id) { Session session = sessionFactory.openSession(); Person person = (Person) session.get(Person.class, id); return person; } public static Person save(Person person) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.save(person); session.getTransaction().commit(); return person; } public static Person update(Person person) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.merge(person); session.getTransaction().commit(); return person; } public static void delete(Person person) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.delete(person); session.getTransaction().commit(); } public static List<Person> findAll(){ Session session = sessionFactory.openSession(); List<Person> persons = session.createQuery("from Person").list(); return persons; } }
Step 9. Create PersonPassportService class: Service class to call the methods of PersonDAO class.
PersonPassportService.java
package com.hibernate.association.service; import java.text.ParseException; import java.text.SimpleDateFormat; import com.hibernate.association.dao.PersonDAO; import com.hibernate.association.pojo.PassportDetail; import com.hibernate.association.pojo.Person; public class PersonPassportService { public static void main(String[] args) { Person person = new Person(); person.setFirstName("Tony"); person.setLastName("Leo"); SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); PassportDetail passportDetail = new PassportDetail(); try { person.setDateOfBirth(sdf.parse("1990-10-10")); passportDetail.setDateOfIssue(sdf.parse("2010-10-10")); passportDetail.setDateOfExpiry(sdf.parse("2020-10-09")); } catch (ParseException e) { e.printStackTrace(); } passportDetail.setPassportNo("Q12345678"); person.setPassportDetail(passportDetail); passportDetail.setPerson(person); // saving person it will generate two insert query. System.out.println(PersonDAO.save(person)); } }
That's it, Now run the PersonPassportService class you will get output at your console where two insert query will executed one for Person and one for PasspportDetail table, along with passport details because we are overriding the toString() method inside Person.java class.
OUT PUT:
Hibernate: insert into person (date_of_birth, first_name, last_name) values (?, ?, ?)
Hibernate: insert into passport_detail (date_of_expiry, date_of_issue, passport_number, person_id) values (?, ?, ?, ?)
Person [personId=1, firstName=Tony, lastName=Leo, dateOfBirth=1990-10-10]
Download the complete example from here Source Code
You can download the same application using hbm.xml without using maven from here one-to-one association using hbm.xml
Sponsored Links
0 comments:
Post a Comment