Sponsored Links
Ad by Google
In my previous post we have seen the example of component mapping using hbm.xml, you can traverse my previous post from Component-Mapping example using hbm.xml. Here we are going to show you the example of component mapping using annotation. Once you create a component that component can be re-use by several places. Here is the text I copied from the documentation "A component is a contained object that is persisted as a value type and not an entity reference. The term "component" refers to the object-oriented notion of composition and not to architecture-level components"
Here is a project table ER diagram we are using for this post.
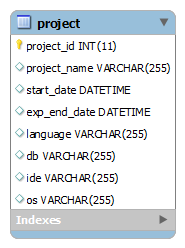
We will create a component class with ide,os,language,db fields.
Overview of the Project Structure:
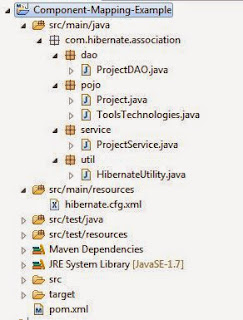
Main Objects of this project are:
Step 1. Create database script.
Step 2. Create a Maven Project:
Step A: Go to File->New->Other..
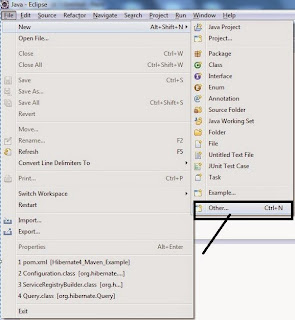
Step B: Select Maven Project from the select wizard.
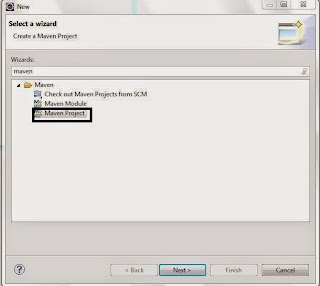
Step C: Select project name and location from New Maven Project wizard.
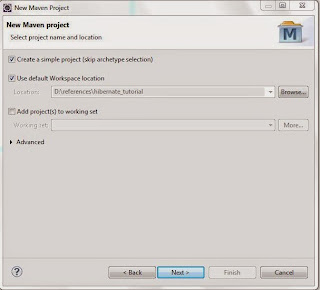
Step D: Configure project, provide GroupId, artifactId etc. See the details from the screenshot. This screen shot is from our previous post so here you need to change the artifact Id as Component-Mapping-Example and project name also as Component-Mapping-Example.
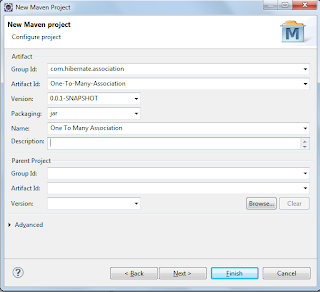
Step E: After completion of all the above steps, now your project will looks like this screenshot.
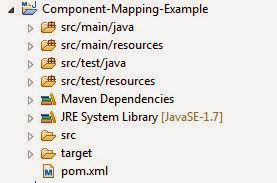
3. Add project dependencies into pom.xml file:
Double click on your project's pom.xml file it will looks like this with very limited information.
Now add Hibernate and MySql dependencies entry inside pom.xml file. Paste the below code inside the project tag of pom.xml file.
Here is a complete pom.xml file
Step 4. Create a hibernate.cfg.xml file: Here we placed hibernate.cfg.xml file inside src/main/resources.
hibernate.cfg.xml
Step 5. Create ToolsTechnologies.java embedable class: Embedable class which will play a role of component class.
ToolsTechnologies.java
Step 6. Create Project.java annotated class.
Project.java
Step 7. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration.
HibernateUtility.java
Step 8. Create ProjectDAO.java class: To perform CRUD operations.
ProjectDAO.java
Step 9. Create ProjectService.java class: To call the methods of ProjectDAO class.
ProjectService.java
That's it. Run the ProjectService.java class and see the output.
OUT PUT:
Language = JAVA
References:
Reference 1
Here is a project table ER diagram we are using for this post.
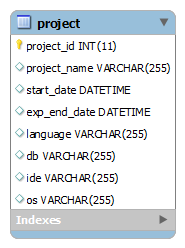
We will create a component class with ide,os,language,db fields.
Tools and Technologies we are using here:
- JDK 7
- Hibernate 4.3.7
- MySql 5.1.10
- Eclipse Juno 4.2
- Maven 3.2
Overview of the Project Structure:
Main Objects of this project are:
- pom.xml
- hibernate.cfg.xml
- annotated pojo
- database
Step 1. Create database script.
CREATE DATABASE /*!32312 IF NOT EXISTS*/`hibernate_tutorial` USE `hibernate_tutorial`; /*Table structure for table `project` */ DROP TABLE IF EXISTS `project`; CREATE TABLE `project` ( `project_id` int(11) NOT NULL AUTO_INCREMENT, `project_name` varchar(255) DEFAULT NULL, `start_date` datetime DEFAULT NULL, `exp_end_date` datetime DEFAULT NULL, `language` varchar(255) DEFAULT NULL, `db` varchar(255) DEFAULT NULL, `ide` varchar(255) DEFAULT NULL, `os` varchar(255) DEFAULT NULL, PRIMARY KEY (`project_id`) ) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=latin1;
Step 2. Create a Maven Project:
Step A: Go to File->New->Other..
Step B: Select Maven Project from the select wizard.
Step C: Select project name and location from New Maven Project wizard.
Step D: Configure project, provide GroupId, artifactId etc. See the details from the screenshot. This screen shot is from our previous post so here you need to change the artifact Id as Component-Mapping-Example and project name also as Component-Mapping-Example.
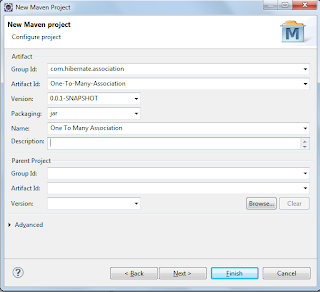
Step E: After completion of all the above steps, now your project will looks like this screenshot.
3. Add project dependencies into pom.xml file:
Double click on your project's pom.xml file it will looks like this with very limited information.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.hibernate.association</groupId> <artifactId>Component-Mapping-Example</artifactId> <version>0.0.1-SNAPSHOT</version> <name>Component-Mapping-Example</name> </project>
Now add Hibernate and MySql dependencies entry inside pom.xml file. Paste the below code inside the project tag of pom.xml file.
<dependencies> <!-- Hibernate Dependency --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>4.3.7.Final</version> </dependency> <!-- MySql Connector dependency --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.10</version> </dependency> </dependencies>
Here is a complete pom.xml file
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.hibernate.association</groupId> <artifactId>Component-Mapping-Example</artifactId> <version>0.0.1-SNAPSHOT</version> <name>Component-Mapping-Example</name> <dependencies> <!-- Hibernate Dependency --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>4.3.7.Final</version> </dependency> <!-- MySql Connector dependency --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.10</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.7</source> <target>1.7</target> </configuration> </plugin> </plugins> </build> </project>
Step 4. Create a hibernate.cfg.xml file: Here we placed hibernate.cfg.xml file inside src/main/resources.
hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/hibernate_tutorial</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <property name="dialect">org.hibernate.dialect.MySQL5InnoDBDialect</property> <property name="show_sql">true</property> <property name="format_sql">true</property> <property name="hbm2ddl.auto">update</property> <mapping class="com.hibernate.association.pojo.Project"/> <mapping class="com.hibernate.association.pojo.ToolsTechnologies"/> </session-factory> </hibernate-configuration>
Step 5. Create ToolsTechnologies.java embedable class: Embedable class which will play a role of component class.
ToolsTechnologies.java
package com.hibernate.association.pojo; import javax.persistence.Column; import javax.persistence.Embeddable; @Embeddable public class ToolsTechnologies { @Column(name = "language") private String language; @Column(name = "db") private String db; @Column(name = "ide") private String ide; @Column(name = "os") private String os; public ToolsTechnologies(String language, String db, String ide, String os) { super(); this.language = language; this.db = db; this.ide = ide; this.os = os; } public ToolsTechnologies() { } public String getLanguage() { return language; } public void setLanguage(String language) { this.language = language; } public String getDb() { return db; } public void setDb(String db) { this.db = db; } public String getIde() { return ide; } public void setIde(String ide) { this.ide = ide; } public String getOs() { return os; } public void setOs(String os) { this.os = os; } }
Step 6. Create Project.java annotated class.
Project.java
package com.hibernate.association.pojo; import java.util.Date; import javax.persistence.Column; import javax.persistence.Embedded; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; import javax.persistence.Temporal; import javax.persistence.TemporalType; @Entity @Table(name = "project") public class Project { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @Column(name = "project_id") private int projectId; @Column(name = "project_name") private String projectName; @Column(name = "start_date") @Temporal(TemporalType.DATE) private Date startDate; @Column(name = "exp_end_date") @Temporal(TemporalType.DATE) private Date expectedEndDate; @Embedded private ToolsTechnologies technologies; public int getProjectId() { return projectId; } public void setProjectId(int projectId) { this.projectId = projectId; } public String getProjectName() { return projectName; } public void setProjectName(String projectName) { this.projectName = projectName; } public Date getStartDate() { return startDate; } public void setStartDate(Date startDate) { this.startDate = startDate; } public Date getExpectedEndDate() { return expectedEndDate; } public void setExpectedEndDate(Date expectedEndDate) { this.expectedEndDate = expectedEndDate; } public ToolsTechnologies getTechnologies() { return technologies; } public void setTechnologies(ToolsTechnologies technologies) { this.technologies = technologies; } @Override public String toString() { return "Project [projectId=" + projectId + ", projectName=" + projectName + ", startDate=" + startDate + ", expectedEndDate=" + expectedEndDate + "]"; } }
Step 7. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration.
HibernateUtility.java
package com.hibernate.association.util; import org.hibernate.SessionFactory; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; public class HibernateUtility { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure(); ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder() .applySettings(configuration.getProperties()).build(); SessionFactory sessionFactory = configuration .buildSessionFactory(serviceRegistry); return sessionFactory; } public static SessionFactory getSessionFactory() { return sessionFactory; } }
Step 8. Create ProjectDAO.java class: To perform CRUD operations.
ProjectDAO.java
package com.hibernate.association.dao; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.hibernate.association.pojo.Project; import com.hibernate.association.util.HibernateUtility; public class ProjectDAO { private static SessionFactory sessionFactory; static { sessionFactory = HibernateUtility.getSessionFactory(); } public static Project findById(int id) { Session session = sessionFactory.openSession(); Project project = (Project) session.load(Project.class, id); return project; } public static Project save(Project project) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.save(project); session.getTransaction().commit(); return project; } public static Project update(Project project) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.merge(project); session.getTransaction().commit(); return project; } public static void delete(Project project) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.delete(project); session.getTransaction().commit(); } }
Step 9. Create ProjectService.java class: To call the methods of ProjectDAO class.
ProjectService.java
package com.hibernate.association.service; import java.text.SimpleDateFormat; import com.hibernate.association.dao.ProjectDAO; import com.hibernate.association.pojo.Project; import com.hibernate.association.pojo.ToolsTechnologies; public class ProjectService { public static void main(String[] args) { try { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); Project project = new Project(); project.setProjectName("MARS"); project.setStartDate(sdf.parse("2012-01-01")); project.setExpectedEndDate(sdf.parse("2016-01-01")); project.setTechnologies(new ToolsTechnologies("JAVA", "MYSQL", "Eclipse Luna", "Linux Ubuntu")); ProjectDAO.save(project); project = ProjectDAO.findById(project.getProjectId()); System.out.println("Project = "+project.getProjectName()); System.out.println("Language = "+project.getTechnologies().getLanguage()); } catch (Exception e) { e.printStackTrace(); } } }
That's it. Run the ProjectService.java class and see the output.
OUT PUT:
Hibernate: insert into project (project_name, start_date, exp_end_date, language, db, ide, os) values (?, ?, ?, ?, ?, ?, ?) Hibernate: select project0_.project_id as project_1_0_0_, project0_.project_name as project_2_0_0_, project0_.start_date as start_da3_0_0_, project0_.exp_end_date as exp_end_4_0_0_, project0_.language as language5_0_0_, project0_.db as db6_0_0_, project0_.ide as ide7_0_0_, project0_.os as os8_0_0_ from project project0_ where project0_.project_id=?Project = MARS
Language = JAVA
Download the complete example from here Source Code
References:
Reference 1
Sponsored Links
Great
ReplyDeleteGood to hear that, you liked it.
ReplyDelete