Sponsored Links
Ad by Google
This article show you how to use Many-To-Many association in hibernate using hbm.xml mapping. For Many-To-Many association example using annotation you can visit my previous post Many-To-Many association example using annotation.
Many-To-Many association means, One or more row(s) of a table are associated with one or more row(s) in another table. For example an employee may assigned with multiple projects, and a project is associated with multiple employees.
In this post we are going to show you the same employee and project association using hbm.xml.
Here is Many-To-Many association mapping table ER diagram.
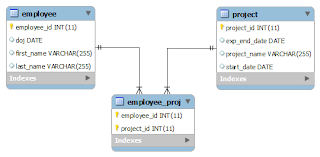
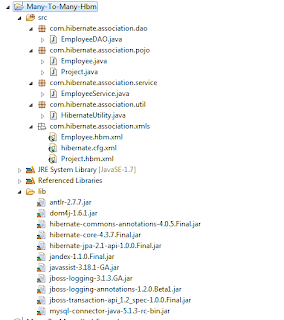
Main Objects of this project are:
Step 1. Create database script.
Step 2. Create a Java Project
Go to File Menu then New->Java Project, and provide the name of the project.
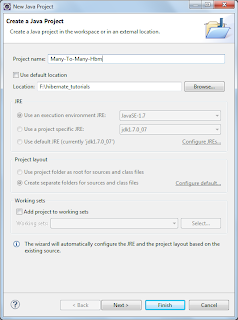
Create a lib folder(Optional):
Right click on your project and create a new folder named it as lib,this step is optional this is just to place all the required jar file. You may put all the jar files anywhere in your system's location, In this project we have placed all the required jar files in lib folder.
Step 3. Add libraries(jar files) into project's build path:
required jar files:
hibernate.cfg.xml
Step 5. Create Employee.hbm.xml file: Here we placed all the xml files inside com.hibernate.association.xmls package
Employee.hbm.xml
Step 6. Create Project.hbm.xml file: Create a mapping file for project table inside com.hibernate.association.xmls package.
Project.hbm.xml
Step 7: Create Employee.java pojo class: It has all the properties defined inside the Employee.hbm.xml file.
Employee.java
Step 8: Create Project.java pojo class.
Project.java
Step 9. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration.
HibernateUtility.java
Step 10: Create EmployeeDAO class: EmployeeDAO class to perform insert/update/get/delete records into database.
EmployeeDAO.java
Step 11. Create an EmployeeService class: EmployeeService class to call the methods of EmployeeDAO class.
EmployeeService.java
That's it. Run EmployeeService and see the output, one insert query for employee two insert query for project and two for employee_proj table.
OUT PUT:
String message printed by toString() method of Employee class,
Employee [employeeId=101, firstName=Tony, lastName=Jha, doj=Thu Aug 28 00:00:00 IST 2014, projects=[Project [projectId=102, projectName=SBA, startDate=Thu Oct 02 00:00:00 IST 2014, expectedEndDate=Mon Jul 04 00:00:00 IST 2016, employees=null], Project [projectId=101, projectName=MARS, startDate=Tue Jan 01 00:00:00 IST 2013, expectedEndDate=Fri Aug 28 00:00:00 IST 2015, employees=null]]]
Many-To-Many association means, One or more row(s) of a table are associated with one or more row(s) in another table. For example an employee may assigned with multiple projects, and a project is associated with multiple employees.
In this post we are going to show you the same employee and project association using hbm.xml.
Here is Many-To-Many association mapping table ER diagram.
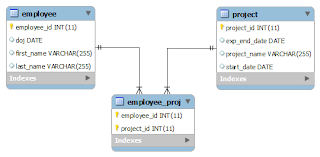
Tools and Technologies we are using here:
- JDK 7
- Hibernate 4.3.7
- MySql 5.1.10
- Eclipse Juno 4.2
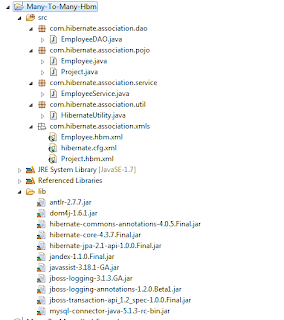
Main Objects of this project are:
- jar files
- hibernate.cfg.xml
- *.hbm.xml(mapping files)
- database
Step 1. Create database script.
CREATE DATABASE /*!32312 IF NOT EXISTS*/`hibernate_tutorial` USE `hibernate_tutorial`; /*Table structure for table `employee` */ DROP TABLE IF EXISTS `employee`; CREATE TABLE `employee` ( `employee_id` int(11) NOT NULL AUTO_INCREMENT, `doj` date DEFAULT NULL, `first_name` varchar(255) DEFAULT NULL, `last_name` varchar(255) DEFAULT NULL, PRIMARY KEY (`employee_id`) ) ENGINE=InnoDB AUTO_INCREMENT=101 DEFAULT CHARSET=latin1; /*Table structure for table `project` */ DROP TABLE IF EXISTS `project`; CREATE TABLE `project` ( `project_id` int(11) NOT NULL AUTO_INCREMENT, `exp_end_date` date DEFAULT NULL, `project_name` varchar(255) DEFAULT NULL, `start_date` date DEFAULT NULL, PRIMARY KEY (`project_id`) ) ENGINE=InnoDB AUTO_INCREMENT=101 DEFAULT CHARSET=latin1;
Step 2. Create a Java Project
Go to File Menu then New->Java Project, and provide the name of the project.
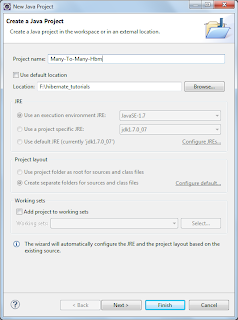
Create a lib folder(Optional):
Right click on your project and create a new folder named it as lib,this step is optional this is just to place all the required jar file. You may put all the jar files anywhere in your system's location, In this project we have placed all the required jar files in lib folder.
Step 3. Add libraries(jar files) into project's build path:
required jar files:
- antlr-2.7.7.jar
- dom4j-1.6.1.jar
- hibernate-commons-annotations-4.0.5.Final.jar
- hibernate-core-4.3.7.Final.jar
- hibernate-jpa-2.1-api-1.0.0.Final.jar
- jandex-1.1.0.Final.jar
- javassist-3.18.1-GA.jar
- jboss-logging-3.1.3.GA.jar
- jboss-logging-annotations-1.2.0.Beta1.jar
- jboss-transaction-api_1.2_spec-1.0.0.Final.jar
- mysql-connector-java-5.1.3-rc-bin.jar
hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/hibernate_tutorial</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <property name="dialect">org.hibernate.dialect.MySQL5InnoDBDialect</property> <property name="show_sql">true</property> <property name="format_sql">true</property> <property name="hbm2ddl.auto">update</property> <mapping resource="com/hibernate/association/xmls/Employee.hbm.xml"/> <mapping resource="com/hibernate/association/xmls/Project.hbm.xml"/> </session-factory> </hibernate-configuration>
Step 5. Create Employee.hbm.xml file: Here we placed all the xml files inside com.hibernate.association.xmls package
Employee.hbm.xml
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="com.hibernate.association.pojo.Employee" table="employee" > <id name="employeeId" type="java.lang.Integer"> <column name="employee_id" /> <generator class="identity" /> </id> <property name="firstName" column="first_name"/> <property name="lastName" column="last_name"/> <property name="doj" column="doj" type="java.util.Date"/> <set name="projects" table="employee_proj" inverse="false" lazy="true" fetch="select" cascade="all" > <key> <column name="employee_id" not-null="true" /> </key> <many-to-many class="com.hibernate.association.pojo.Project"> <column name="project_id" not-null="true" /> </many-to-many> </set> </class> </hibernate-mapping>
Step 6. Create Project.hbm.xml file: Create a mapping file for project table inside com.hibernate.association.xmls package.
Project.hbm.xml
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="com.hibernate.association.pojo.Project" table="project" > <id name="projectId" type="java.lang.Integer"> <column name="project_id" /> <generator class="identity" /> </id> <property name="projectName" column="project_name"/> <property name="startDate" column="start_date" type="java.util.Date"/> <property name="expEndDate" column="exp_end_date" type="java.util.Date"/> <set name="employees" table="employee_proj" inverse="true" lazy="true" fetch="select"> <key> <column name="project_id" not-null="true" /> </key> <many-to-many class="com.hibernate.association.pojo.Employee"> <column name="employee_id" not-null="true" /> </many-to-many> </set> </class> </hibernate-mapping>
Step 7: Create Employee.java pojo class: It has all the properties defined inside the Employee.hbm.xml file.
Employee.java
package com.hibernate.association.pojo; import java.util.Date; import java.util.Set; public class Employee { private int employeeId; private String firstName; private String lastName; private Date doj; private Set<Project> projects; public int getEmployeeId() { return employeeId; } public void setEmployeeId(int employeeId) { this.employeeId = employeeId; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public Date getDoj() { return doj; } public void setDoj(Date doj) { this.doj = doj; } public Set<Project> getProjects() { return projects; } public void setProjects(Set<Project> projects) { this.projects = projects; } @Override public String toString() { return "Employee [employeeId=" + employeeId + ", firstName=" + firstName + ", lastName=" + lastName + ", doj=" + doj + ", projects=" + projects + "]"; } }
Step 8: Create Project.java pojo class.
Project.java
package com.hibernate.association.pojo; import java.util.Date; import java.util.Set; public class Project { private int projectId; private String projectName; private Date startDate; private Date expEndDate; private Set<Employee> employees; public int getProjectId() { return projectId; } public void setProjectId(int projectId) { this.projectId = projectId; } public String getProjectName() { return projectName; } public void setProjectName(String projectName) { this.projectName = projectName; } public Date getStartDate() { return startDate; } public void setStartDate(Date startDate) { this.startDate = startDate; } public Date getExpEndDate() { return expEndDate; } public void setExpEndDate(Date expEndDate) { this.expEndDate = expEndDate; } public Set<Employee> getEmployees() { return employees; } public void setEmployees(Set<Employee> employees) { this.employees = employees; } @Override public String toString() { return "Project [projectId=" + projectId + ", projectName=" + projectName + ", startDate=" + startDate + ", expEndDate=" + expEndDate + ", employees=" + employees + "]"; } }
Step 9. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration.
HibernateUtility.java
package com.hibernate.association.util; import org.hibernate.SessionFactory; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; public class HibernateUtility { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure("/com/hibernate/association/xmls/hibernate.cfg.xml"); ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder() .applySettings(configuration.getProperties()).build(); SessionFactory sessionFactory = configuration .buildSessionFactory(serviceRegistry); return sessionFactory; } public static SessionFactory getSessionFactory() { return sessionFactory; } }
Step 10: Create EmployeeDAO class: EmployeeDAO class to perform insert/update/get/delete records into database.
EmployeeDAO.java
package com.hibernate.association.dao; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.hibernate.association.pojo.Employee; import com.hibernate.association.util.HibernateUtility; public class EmployeeDAO { private static SessionFactory sessionFactory; static { sessionFactory = HibernateUtility.getSessionFactory(); } public static Employee findById(int id) { Session session = sessionFactory.openSession(); Employee employee = (Employee) session.load(Employee.class, id); return employee; } public static Employee save(Employee employee) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.save(employee); session.getTransaction().commit(); return employee; } public static Employee update(Employee employee) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.merge(employee); session.getTransaction().commit(); return employee; } public static void delete(Employee employee) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.delete(employee); session.getTransaction().commit(); } }
Step 11. Create an EmployeeService class: EmployeeService class to call the methods of EmployeeDAO class.
EmployeeService.java
package com.hibernate.association.service; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.HashSet; import java.util.Set; import com.hibernate.association.dao.EmployeeDAO; import com.hibernate.association.pojo.Employee; import com.hibernate.association.pojo.Project; public class EmployeeService { public static void main(String[] args) { Employee employee = new Employee(); SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); try { // employee details employee.setDoj(sdf.parse("2014-08-28")); employee.setFirstName("Tony"); employee.setLastName("Jha"); // project details Project mars = new Project(); mars.setProjectName("MARS"); mars.setStartDate(sdf.parse("2013-01-01")); mars.setExpEndDate(sdf.parse("2015-08-28")); // project 2 details Project sba = new Project(); sba.setProjectName("SBA"); sba.setStartDate(sdf.parse("2014-10-02")); sba.setExpEndDate(sdf.parse("2016-07-04")); Set<Project> projects = new HashSet<>(); projects.add(mars); projects.add(sba); employee.setProjects(projects); // calling save method of EmployeeDAO System.out.println(EmployeeDAO.save(employee)); } catch (ParseException e) { e.printStackTrace(); } } }
That's it. Run EmployeeService and see the output, one insert query for employee two insert query for project and two for employee_proj table.
OUT PUT:
Hibernate: insert into employee (doj, first_name, last_name) values (?, ?, ?) Hibernate: insert into project (exp_end_date, project_name, start_date) values (?, ?, ?) Hibernate: insert into project (exp_end_date, project_name, start_date) values (?, ?, ?) Hibernate: insert into employee_proj (employee_id, project_id) values (?, ?) Hibernate: insert into employee_proj (employee_id, project_id) values (?, ?)
String message printed by toString() method of Employee class,
Employee [employeeId=101, firstName=Tony, lastName=Jha, doj=Thu Aug 28 00:00:00 IST 2014, projects=[Project [projectId=102, projectName=SBA, startDate=Thu Oct 02 00:00:00 IST 2014, expectedEndDate=Mon Jul 04 00:00:00 IST 2016, employees=null], Project [projectId=101, projectName=MARS, startDate=Tue Jan 01 00:00:00 IST 2013, expectedEndDate=Fri Aug 28 00:00:00 IST 2015, employees=null]]]
Download the complete example from here Source Code
Sponsored Links
You are doing great job.. Keep it up.. :)
ReplyDeleteThanks Dear :) keep visiting definitely you will find something interesting.
ReplyDelete