Sponsored Links
Ad by Google
In this post we are going to implement the component mapping, component is again one of the important feature of hibernate, once you create a component you can re-use this component on several places where required. In this post we are implementing component mapping using hbm.xml file.
Here is a project table ER diagram we are using for this post.
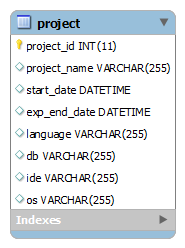

Main Objects of this project are:
Step 2. Create a Java Project
Go to File Menu then New->Java Project, and provide the name of the project.

Create a lib folder(Optional):
Right click on your project and create a new folder named it as lib,this step is optional this is just to place all the required jar file. You may put all the jar files anywhere in your system's location, In this project we have placed all the required jar files in lib folder.
Step 3. Add libraries(jar files) into project's build path:
required jar files:
Step 4. Create a hibernate.cfg.xml file: Here we placed hibernate.cfg.xml file inside com.hibernate.association.xmls package.
hibernate.cfg.xml
Step 5. Create Project.hbm.xml mapping file: Here we placed all the xml files inside com.hibernate.association.xmls package.
Project.hbm.xml
Step 6: Create Project.java pojo class: It contains all the properties defined inside the project.hbm.xml mapping file.
Project.java
Step 7: Create ToolsTechnologies.java class: ToolsTechnologies is a component class contains component properties like language,db,os etc. We use this class as a property of Project.java class.
ToolsTechnologies.java
Step 8. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration.
HibernateUtility.java
Step 9: Create ProjectDAO.java class: ProjectDAO class to perform CRUD operations.
ProjectDAO.java
Step 10: Create ProjectService.java class: To call the methods of ProjectDAO class.
ProjectService.java
OUT PUT:
Language = JAVA
References:
Reference 1
Here is a project table ER diagram we are using for this post.
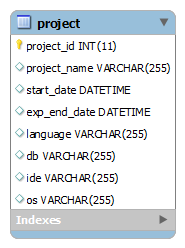
Tools and Technologies we are using here:
- JDK 7
- Hibernate 4.3.7
- MySql 5.1.10
- Eclipse Juno 4.2

Main Objects of this project are:
- jar files
- hibernate.cfg.xml
- *.hbm.xml(mapping files)
- database
CREATE DATABASE /*!32312 IF NOT EXISTS*/`hibernate_tutorial` USE `hibernate_tutorial`; /*Table structure for table `project` */ DROP TABLE IF EXISTS `project`; CREATE TABLE `project` ( `project_id` int(11) NOT NULL AUTO_INCREMENT, `project_name` varchar(255) DEFAULT NULL, `start_date` datetime DEFAULT NULL, `exp_end_date` datetime DEFAULT NULL, `language` varchar(255) DEFAULT NULL, `db` varchar(255) DEFAULT NULL, `ide` varchar(255) DEFAULT NULL, `os` varchar(255) DEFAULT NULL, PRIMARY KEY (`project_id`) ) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=latin1;
Step 2. Create a Java Project
Go to File Menu then New->Java Project, and provide the name of the project.

Create a lib folder(Optional):
Right click on your project and create a new folder named it as lib,this step is optional this is just to place all the required jar file. You may put all the jar files anywhere in your system's location, In this project we have placed all the required jar files in lib folder.
Step 3. Add libraries(jar files) into project's build path:
required jar files:
- antlr-2.7.7.jar
- dom4j-1.6.1.jar
- hibernate-commons-annotations-4.0.5.Final.jar
- hibernate-core-4.3.7.Final.jar
- hibernate-jpa-2.1-api-1.0.0.Final.jar
- jandex-1.1.0.Final.jar
- javassist-3.18.1-GA.jar
- jboss-logging-3.1.3.GA.jar
- jboss-logging-annotations-1.2.0.Beta1.jar
- jboss-transaction-api_1.2_spec-1.0.0.Final.jar
- mysql-connector-java-5.1.3-rc-bin.jar
Step 4. Create a hibernate.cfg.xml file: Here we placed hibernate.cfg.xml file inside com.hibernate.association.xmls package.
hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/hibernate_tutorial</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <property name="dialect">org.hibernate.dialect.MySQL5InnoDBDialect</property> <property name="show_sql">true</property> <property name="format_sql">true</property> <property name="hbm2ddl.auto">update</property> <mapping resource="com/hibernate/association/xmls/Project.hbm.xml"/> </session-factory> </hibernate-configuration>
Step 5. Create Project.hbm.xml mapping file: Here we placed all the xml files inside com.hibernate.association.xmls package.
Project.hbm.xml
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="com.hibernate.association.pojo.Project" table="project" > <id name="projectId" type="java.lang.Integer"> <column name="project_id" /> <generator class="identity" /> </id> <property name="projectName" column="project_name"/> <property name="startDate" column="start_date" type="java.util.Date"/> <property name="expectedEndDate" column="exp_end_date" type="java.util.Date"/> <component name="toolsTechnologies" class="com.hibernate.association.pojo.ToolsTechnologies"> <property name="language" column="language" /> <property name="db" column="db"/> <property name="ide" column="ide"/> <property name="os" column="os"/> </component> </class> </hibernate-mapping>
Step 6: Create Project.java pojo class: It contains all the properties defined inside the project.hbm.xml mapping file.
Project.java
package com.hibernate.association.pojo; import java.util.Date; public class Project { private int projectId; private String projectName; private Date startDate; private Date expectedEndDate; private ToolsTechnologies toolsTechnologies; public int getProjectId() { return projectId; } public void setProjectId(int projectId) { this.projectId = projectId; } public String getProjectName() { return projectName; } public void setProjectName(String projectName) { this.projectName = projectName; } public Date getStartDate() { return startDate; } public void setStartDate(Date startDate) { this.startDate = startDate; } public Date getExpectedEndDate() { return expectedEndDate; } public void setExpectedEndDate(Date expectedEndDate) { this.expectedEndDate = expectedEndDate; } public ToolsTechnologies getToolsTechnologies() { return toolsTechnologies; } public void setToolsTechnologies(ToolsTechnologies toolsTechnologies) { this.toolsTechnologies = toolsTechnologies; } @Override public String toString() { return "Project [projectId=" + projectId + ", projectName=" + projectName + ", startDate=" + startDate + ", expectedEndDate=" + expectedEndDate + "]"; } }
Step 7: Create ToolsTechnologies.java class: ToolsTechnologies is a component class contains component properties like language,db,os etc. We use this class as a property of Project.java class.
ToolsTechnologies.java
package com.hibernate.association.pojo; public class ToolsTechnologies { private String language; private String db; private String ide; private String os; public ToolsTechnologies() { } public ToolsTechnologies(String language, String db, String ide, String os) { super(); this.language = language; this.db = db; this.ide = ide; this.os = os; } public String getLanguage() { return language; } public void setLanguage(String language) { this.language = language; } public String getDb() { return db; } public void setDb(String db) { this.db = db; } public String getIde() { return ide; } public void setIde(String ide) { this.ide = ide; } public String getOs() { return os; } public void setOs(String os) { this.os = os; } }
Step 8. Create a HibernateUtility class: HibernateUtility class to build SessionFactory via loading Configuration.
HibernateUtility.java
package com.hibernate.association.util; import org.hibernate.SessionFactory; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; public class HibernateUtility { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure("/com/hibernate/association/xmls/hibernate.cfg.xml"); ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder() .applySettings(configuration.getProperties()).build(); SessionFactory sessionFactory = configuration .buildSessionFactory(serviceRegistry); return sessionFactory; } public static SessionFactory getSessionFactory() { return sessionFactory; } }
Step 9: Create ProjectDAO.java class: ProjectDAO class to perform CRUD operations.
ProjectDAO.java
package com.hibernate.association.dao; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.hibernate.association.pojo.Project; import com.hibernate.association.util.HibernateUtility; public class ProjectDAO { private static SessionFactory sessionFactory; static { sessionFactory = HibernateUtility.getSessionFactory(); } public static Project findById(int id) { Session session = sessionFactory.openSession(); Project project = (Project) session.load(Project.class, id); return project; } public static Project save(Project project) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.save(project); session.getTransaction().commit(); return project; } public static Project update(Project project) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.merge(project); session.getTransaction().commit(); return project; } public static void delete(Project project) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.delete(project); session.getTransaction().commit(); } }
Step 10: Create ProjectService.java class: To call the methods of ProjectDAO class.
ProjectService.java
package com.hibernate.association.service; import java.text.SimpleDateFormat; import com.hibernate.association.dao.ProjectDAO; import com.hibernate.association.pojo.Project; import com.hibernate.association.pojo.ToolsTechnologies; public class ProjectService { public static void main(String[] args) { try { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); Project project = new Project(); project.setProjectName("MARS"); project.setStartDate(sdf.parse("2012-01-01")); project.setExpectedEndDate(sdf.parse("2016-01-01")); project.setToolsTechnologies(new ToolsTechnologies("JAVA", "MYSQL", "Eclipse Luna", "Linux Ubuntu")); ProjectDAO.save(project); project = ProjectDAO.findById(project.getProjectId()); System.out.println("Project = "+project.getProjectName()); System.out.println("Language = "+project.getToolsTechnologies().get Language()); } catch (Exception e) { e.printStackTrace(); } } }
OUT PUT:
Hibernate: insert into project (project_name, start_date, exp_end_date, language, db, ide, os) values (?, ?, ?, ?, ?, ?, ?) Hibernate: select project0_.project_id as project_1_0_0_, project0_.project_name as project_2_0_0_, project0_.start_date as start_da3_0_0_, project0_.exp_end_date as exp_end_4_0_0_, project0_.language as language5_0_0_, project0_.db as db6_0_0_, project0_.ide as ide7_0_0_, project0_.os as os8_0_0_ from project project0_ where project0_.project_id=?Project = MARS
Language = JAVA
Download the complete example from here Source Code
References:
Reference 1
Sponsored Links
0 comments:
Post a Comment