Sponsored Links
Ad by Google
In hibernate generator classes are used to generate unique identifiers for instances of the persistence class. Hibernate provides the list of built in generator classes to generate unique identifiers, all the generator classes implements the org.hibernate.id.IdentifierGenerator interface, and if your needs of unique identifiers is not solved by using built in generator classes, then you can create your own generator classes by implementing org.hibernate.id.IdentifierGenerator interface. Here is a example of custom generator class via implementing org.hibernate.id.IdentifierGenerator interface.
In this post we are going to show you the implementation of identity generator, one of my favorite generator provided by hibernate. The identity generator is not supported by all the databases like oracle database does not support this, but DB2, MySQL, MS SQL Server, Sybase and HypersonicSQL supports identity generator.
If no <generator> element is specified, then hibernate will assign the default generator as assigned, assigned is the default generator provided by hibernate here is the implementation of default generator strategy in hibernate.
Here is our generator table ER-Diagram, we are using for this project.
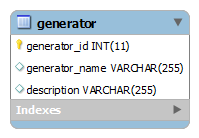

Main Objects of this project are:
Step 1. Create database, here is the create script for the table and database.
Step 2. Create a maven project and add the below given dependencies for hibernate and mysql, inside your pom.xml file.
Step 3. Create a hibernate.cfg.xml file inside src/main/resources folder.
hibernate.cfg.xml
Step 4. Create Generator.hbm.xml mapping file inside src/main/resources folder. Here the child element of id generator element is identity.
Generator.hbm.xml
Step 5. Create HibernateUtility.java class to return SessionFactory in hibernate 4.3.7
HibernateUtility.java
Step 6. Create Generator.java pojo class, It has all the properties mapped inside the Generator.hbm.xml file.
Generator
Step 7. Create GeneratorDAO.java class and provide the implementation of CRUD method.
GeneratorDAO
Step 8. Create GeneratorService.java class to call the methods of GeneratorDAO class.
GeneratorService
Run the GeneratorService, It will create a new record inside the generator table of hibernate_tutorial database.
OUT PUT:
Hibernate: insert into generator (generator_name, description, generator_id) values (?, ?, ?)
Generator [generatorId=1, generatorName=IDENTITY, description=IDENTITY generator class used]
Download the complete example from here Source Code
References:
Reference 1
Reference 2
In this post we are going to show you the implementation of identity generator, one of my favorite generator provided by hibernate. The identity generator is not supported by all the databases like oracle database does not support this, but DB2, MySQL, MS SQL Server, Sybase and HypersonicSQL supports identity generator.
If no <generator> element is specified, then hibernate will assign the default generator as assigned, assigned is the default generator provided by hibernate here is the implementation of default generator strategy in hibernate.
Here is our generator table ER-Diagram, we are using for this project.
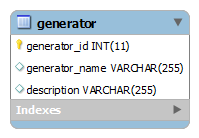
Tools and Technologies we are using here:
- JDK 7
- Hibernate 4.3.7
- MySql 5.1.10
- Eclipse Juno 4.2
- Maven 3.2

Main Objects of this project are:
- pom.xml
- hibernate.cfg.xml
- Generator.hbm.xml
- database
Step 1. Create database, here is the create script for the table and database.
CREATE DATABASE /*!32312 IF NOT EXISTS*/`hibernate_tutorial` USE `hibernate_tutorial`; /*Table structure for table `generator` */ DROP TABLE IF EXISTS `generator`; CREATE TABLE `generator` ( `generator_id` int(11) NOT NULL AUTO_INCREMENT, `generator_name` varchar(255) DEFAULT NULL, `description` varchar(255) DEFAULT NULL, PRIMARY KEY (`generator_id`) ) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=latin1;
Step 2. Create a maven project and add the below given dependencies for hibernate and mysql, inside your pom.xml file.
<dependencies> <!-- Hibernate Dependency --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>4.3.7.Final</version> </dependency> <!-- MySql Connector dependency --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.10</version> </dependency> </dependencies>
Step 3. Create a hibernate.cfg.xml file inside src/main/resources folder.
hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/hibernate_tutorial</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <!-- SQL dialect --> <property name="dialect">org.hibernate.dialect.MySQLDialect</property> <!-- Echo all executed SQL to stdout --> <property name="show_sql">true</property> <property name="hbm2ddl.auto">update</property> <mapping resource="Generator.hbm.xml"/> </session-factory> </hibernate-configuration>
Step 4. Create Generator.hbm.xml mapping file inside src/main/resources folder. Here the child element of id generator element is identity.
Generator.hbm.xml
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <!-- Generated 29 Oct, 2014 8:47:37 PM by Hibernate Tools 3.4.0.CR1 --> <hibernate-mapping> <class name="com.hibernate.generator.pojo.Generator" table="generator"> <id name="generatorId" type="java.lang.Integer" column="generator_id" > <generator class="identity"/><!-- Here we are using identity --> </id> <property name="generatorName" column="generator_name"/> <property name="description" column="description"/> </class> </hibernate-mapping>
Step 5. Create HibernateUtility.java class to return SessionFactory in hibernate 4.3.7
HibernateUtility.java
package com.hibernate.generator.util; import org.hibernate.SessionFactory; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; public class HibernateUtility { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure(); ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder() .applySettings(configuration.getProperties()).build(); SessionFactory sessionFactory = configuration .buildSessionFactory(serviceRegistry); return sessionFactory; } public static SessionFactory getSessionFactory() { return sessionFactory; } }
Step 6. Create Generator.java pojo class, It has all the properties mapped inside the Generator.hbm.xml file.
Generator
package com.hibernate.generator.pojo; import com.hibernate.generator.util.GeneratorType; public class Generator { private int generatorId; private String generatorName; private String description; public int getGeneratorId() { return generatorId; } public void setGeneratorId(int generatorId) { this.generatorId = generatorId; } public String getGeneratorName() { return generatorName; } public void setGeneratorName(String generatorName) { this.generatorName = generatorName; } public String getDescription() { return description; } public void setDescription(String description) { this.description = description; } @Override public String toString() { return "Generator [generatorId=" + generatorId + ", generatorName=" + generatorName + ", description=" + description + "]"; } }
Step 7. Create GeneratorDAO.java class and provide the implementation of CRUD method.
GeneratorDAO
package com.hibernate.generator.dao; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.hibernate.generator.pojo.Generator; import com.hibernate.generator.util.HibernateUtility; public class GeneratorDAO { private static SessionFactory sessionFactory; static { sessionFactory = HibernateUtility.getSessionFactory(); } public static Generator findById(long id) { Session session = sessionFactory.openSession(); Generator generator = (Generator) session.load(Generator.class, id); return generator; } public static Generator save(Generator generator) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.save(generator); session.getTransaction().commit(); return generator; } public static Generator update(Generator generator) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.merge(generator); session.getTransaction().commit(); return generator; } public static void delete(Generator generator) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.delete(generator); session.getTransaction().commit(); } }
Step 8. Create GeneratorService.java class to call the methods of GeneratorDAO class.
GeneratorService
package com.hibernate.generator.service; import com.hibernate.generator.dao.GeneratorDAO; import com.hibernate.generator.pojo.Generator; import com.hibernate.generator.util.GeneratorType; public class GeneratorService { public static void main(String[] args) { Generator generator = new Generator(); generator.setDescription("IDENTITY generator class used"); generator.setGeneratorName("IDENTITY"); System.out.println(GeneratorDAO.save(generator)); } }
Run the GeneratorService, It will create a new record inside the generator table of hibernate_tutorial database.
OUT PUT:
Hibernate: insert into generator (generator_name, description, generator_id) values (?, ?, ?)
Generator [generatorId=1, generatorName=IDENTITY, description=IDENTITY generator class used]
Download the complete example from here Source Code
References:
Reference 1
Reference 2
Sponsored Links
0 comments:
Post a Comment