Sponsored Links
Ad by Google
In this post we are going to show you, how to create custom generator class in hibernate. In hibernate generator class is used to generate unique identifiers for instances of persistence class. Hibernate provides you the list of default generator classes and of course they all have advantages and disadvantages. But sometimes it may, you need more specialized unique identifiers for your persistence classes, It may that your requirement is not fulfilled by the default generator class and your job is to create your own generator class. For example for one of our project requirement was to create an employee id with the combination of R&D000001,R&D000002..and so on. In this post we are going to implement the same, It will first fetched the latest created employee id from the database and add +1 value to it each time we insert new employee into the database.
Lets create our own generator class and implement in our persistence class. In hibernate all default generator classes are implement org.hibernate.id.IdentifierGenerator interface. So to create our own generator class we also have to implement org.hibernate.id.IdentifierGenerator interface.
Here is our employee table ER diagram.
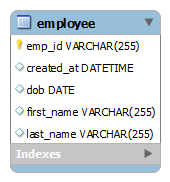
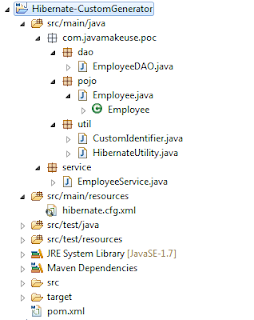
Main Objects of this project are:
Step 1. Create a maven project and add the below given dependencies for hibernate and mysql, inside your pom.xml file.
Step 2. Create a hibernate.cfg.xml file:
Create hibernate.cfg.xml file inside src/main/resources folder.
hibernate.cfg.xml
Step 3. Create CustomIdentifier.java class, our main objective of this project is to create our own generator class. CustomIdentifier class is implementing an interface called IdentifierGenerator and provides the implementation of generate method.
Step 4. Create HibernateUtility.java class to return SessionFactory in hibernate 4.3.7
HibernateUtility.java
Employee.java
Step 6. Create EmployeeDAO.java class to save/delete records.
EmployeeDAO.java
Step 7. Create EmployeeService.java class to call the methods of EmployeeDAO class.
EmployeeService.java
Run EmployeeService.java class, it will insert a record into the employee table, inserted empId would be something like R&D000001,R&D000002 ...R&D000005 and so on.
That's it :)
Here is the complete Hibernate Tutorial, where you can find the implementation of all the features of hibernate
Lets create our own generator class and implement in our persistence class. In hibernate all default generator classes are implement org.hibernate.id.IdentifierGenerator interface. So to create our own generator class we also have to implement org.hibernate.id.IdentifierGenerator interface.
Here is our employee table ER diagram.
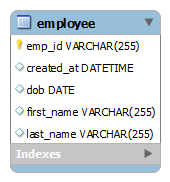
Tools and Technologies we are using here:
- JDK 7
- Hibernate 4.3.7
- MySql 5.1.10
- Eclipse Juno 4.2
- Maven 3.2
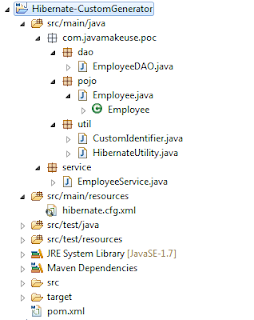
Main Objects of this project are:
- pom.xml
- hibernate.cfg.xml
- annotated pojo
- CustomIdentifier.java
- database
Step 1. Create a maven project and add the below given dependencies for hibernate and mysql, inside your pom.xml file.
<dependencies> <!-- Hibernate Dependency --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>4.3.7.Final</version> </dependency> <!-- MySql Connector dependency --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.10</version> </dependency> </dependencies>
Step 2. Create a hibernate.cfg.xml file:
Create hibernate.cfg.xml file inside src/main/resources folder.
hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/hibernate_tutorial</property> <property name="connection.username">root</property> <property name="connection.password">root</property> <!-- SQL dialect --> <property name="dialect">org.hibernate.dialect.MySQLDialect</property> <!-- Echo all executed SQL to stdout --> <property name="show_sql">true</property> <property name="hbm2ddl.auto">update</property> <mapping class="com.javamakeuse.poc.pojo.Employee"/> </session-factory> </hibernate-configuration>
Step 3. Create CustomIdentifier.java class, our main objective of this project is to create our own generator class. CustomIdentifier class is implementing an interface called IdentifierGenerator and provides the implementation of generate method.
package com.javamakeuse.poc.util; import java.io.Serializable; import java.sql.Connection; import java.sql.ResultSet; import java.sql.SQLException; import org.hibernate.HibernateException; import org.hibernate.engine.spi.SessionImplementor; import org.hibernate.id.IdentifierGenerator; public class CustomIdentifier implements IdentifierGenerator { private String defaultPrefix = "R&D"; private int defaultNumber = 1; @Override public Serializable generate(SessionImplementor session, Object arg1) throws HibernateException { String empId = ""; String digits = ""; Connection con = session.connection(); try { java.sql.PreparedStatement pst = con .prepareStatement("select emp_id from employee order by created_at desc limit 1"); ResultSet rs = pst.executeQuery(); if (rs != null && rs.next()) { empId = rs.getString("emp_id"); System.out.println(empId); String prefix = empId.substring(0, 3); String str[] = empId.split(prefix); digits = String.format("%06d", Integer.parseInt(str[1]) + 1); empId = prefix.concat(digits); } else { digits = String.format("%06d", defaultNumber); empId = defaultPrefix.concat(digits); } } catch (SQLException e) { e.printStackTrace(); } return empId; } }
Step 4. Create HibernateUtility.java class to return SessionFactory in hibernate 4.3.7
HibernateUtility.java
package com.javamakeuse.poc.util; import org.hibernate.SessionFactory; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; public class HibernateUtility { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { Configuration configuration = new Configuration(); configuration.configure(); ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder() .applySettings(configuration.getProperties()).build(); SessionFactory sessionFactory = configuration .buildSessionFactory(serviceRegistry); return sessionFactory; } public static SessionFactory getSessionFactory() { return sessionFactory; } }Step 5. Create Employee.java annotated classes
Employee.java
package com.javamakeuse.poc.pojo; import java.util.Date; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.persistence.Table; import javax.persistence.Temporal; import javax.persistence.TemporalType; import org.hibernate.annotations.GenericGenerator; @Entity @Table(name = "employee") public class Employee { @Id @GenericGenerator(name = "custom_id", strategy = "com.javamakeuse.poc.util.CustomIdentifier") @GeneratedValue(generator = "custom_id") @Column(name = "emp_id") private String empId; @Column(name = "first_name") private String firstName; @Column(name = "last_name") private String lastName; @Temporal(TemporalType.DATE) @Column(name = "dob") private Date dob; @Temporal(TemporalType.TIMESTAMP) @Column(name = "created_at") private Date createdAt; public String getEmpId() { return empId; } public void setEmpId(String empId) { this.empId = empId; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public Date getDob() { return dob; } public void setDob(Date dob) { this.dob = dob; } public Date getCreatedAt() { return createdAt; } public void setCreatedAt(Date createdAt) { this.createdAt = createdAt; } }
Step 6. Create EmployeeDAO.java class to save/delete records.
EmployeeDAO.java
package com.javamakeuse.poc.dao; import org.hibernate.Session; import org.hibernate.SessionFactory; import com.javamakeuse.poc.pojo.Employee; import com.javamakeuse.poc.util.HibernateUtility; public class EmployeeDAO { private static SessionFactory sessionFactory; static { sessionFactory = HibernateUtility.getSessionFactory(); } public static Employee save(Employee employee) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.save(employee); session.getTransaction().commit(); return employee; } public static void delete(Employee employee) { Session session = sessionFactory.openSession(); session.beginTransaction(); session.delete(employee); session.getTransaction().commit(); } }
Step 7. Create EmployeeService.java class to call the methods of EmployeeDAO class.
EmployeeService.java
package service; import java.text.ParseException; import java.util.Calendar; import com.javamakeuse.poc.dao.EmployeeDAO; import com.javamakeuse.poc.pojo.Employee; public class EmployeeService { public static void main(String[] args) throws ParseException { Employee employee = new Employee(); employee.setCreatedAt(Calendar.getInstance().getTime()); employee.setDob(Calendar.getInstance().getTime()); employee.setFirstName("Vi"); employee.setLastName("Jha"); EmployeeDAO.save(employee); } }
Run EmployeeService.java class, it will insert a record into the employee table, inserted empId would be something like R&D000001,R&D000002 ...R&D000005 and so on.
That's it :)
Here is the complete Hibernate Tutorial, where you can find the implementation of all the features of hibernate
Download the complete example from here Source Code
Sponsored Links
0 comments:
Post a Comment